We have already covered the basic concepts of using arrays and understand how to implement an array. String is a character array as well as linear data structure ( we follow a sequence of characters in the array data structure ).
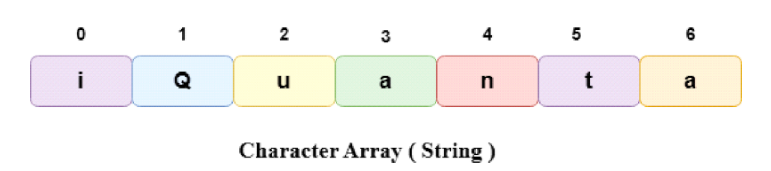
There are various examples of using string in data structures like spelling checkers : if we want to check the spellings in a text or paragraph, as a database engineer if I want to check the valid information of a user in a database, and many more.
In this blog we will cover the introduction of string in data structure, basic operations performed on a string, analyzing complexities of operations performed on a string in data structure, application of using string in a data structure, various advantages and disadvantages of using string in data structure.
What is String in Data Structure?
String is a linear data structure and it is also known as the character array ( array of characters). We are sequentially storing characters in the string like the way we are doing in the integer arrays. String data structure is having a null character ‘\0’ that terminates the entire string in data structure.
Here is the pictorial representation for understanding string in data structure and the working of it :
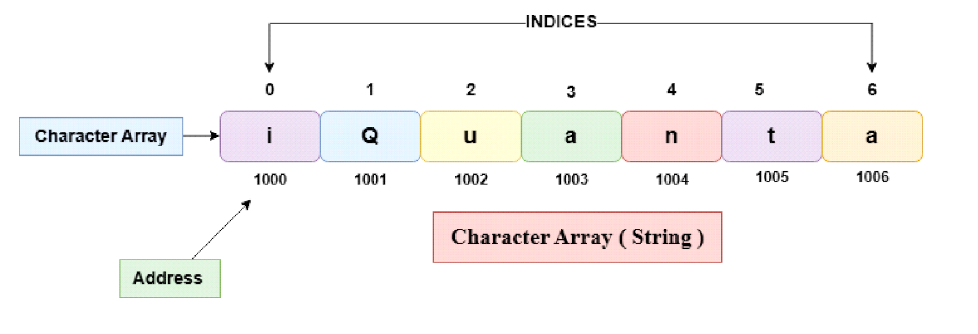
Representation of string in data structures in memory is the representation of an array of characters. For every character in the array there is a unique index associated with it.
Unique indices help to access characters from the string so we can perform multiple operations on it like modification , searching of character, deletion of character in the string and concatenation as well.
Example : “ Hello “ itself is a string that consists of 5 characters.
Strings in a programming language generally represented by something written inside the double inverted commas. It is a fundamental concept to understand text and file handling in data structures or in different programming languages.
There are different built- in functions or keyword that we are using to analyze string in a data structure includes :
sizeof ( ) function
sizeof ( ) function in string used to find the size of the string, size of ( ) function counts the null character also in the string and returns the count value in the form of integer.
Example : char arr_name [ 24 ] = “ iQuanta iskills” ;
Value printed : 16 ( includes the null character )
strlen( ) function
strlen( ) function is a very useful function of string data structures that calculates the length of the string but it does not count the null character ( terminated character of the string ) to return the calculated value of the string length in integer form.;
Example : char arr_name [ 24 ] = “iQuanta iskills”;
Value printed : 15 ( it doesn’t includes the null character )
String Operations
There are different operations we can perform in a data structure along with an examples :
Insertion Operation
Inserting characters in a given string name str_iskills at a specified position .
Example : char str_iskills [ 18 ]= “ iskills iQuant”
In this example I want to insert an element ( or character ) at the last index ‘14’. Let’s take a character ‘a’ that we need to add in the original character string , the respective output of the operation is specified below.
Char str_iskills [ 18 ] =”iskills iQuanta”;
‘a’ is added at the end of the character array which is required to add at the specified index.
Access Operation
Access operation in string is used to access a particular character of a string by referring to the index number.
Example : char arr_iskills [ 18 ] = “ iskills iQuanta”;
If i want to access ‘ Q ‘ from the string then we have to specify it to the index number to find or access the character from the string.
Deletion Operation
Deletion operation in a string is used to delete the particular character from the string ( array of characters ).
Example : char arr_iskills [ 20 ] = “iskillss iQuanta “;
Here in this example I want to delete the extra ‘s’ from the iskills. In this case i have to use the delete operation to remove the extra character from the string.
The final output for the deletion operation specified below :
char arr_iskills [ 20 ] = “iskills iQuanta”;
Concatenation Operation
Concatenation operation performed on the string to join the string together. If we take a real life application that we want to show or display the full name of a person then in this case we have to concatenate the first name and the last name of a person to get the full name.
Example : char arr_string1 [ 10 ] = “ iskills” ;
char arr_string2 [ 20 ] =”iQuanta”;
We need to join both the strings arr_string1 and arr_string2 while performing concatenation operations.
Specified output : char output_string [ 40 ] = “iskills iQuanta”;
( “Iskills iQuanta” ) specified output of the concatenated string.
Complexity Analysis of String in Data Structure
Analyzing complexity of string in data structure based on the operations performed in the string data structure. While performing algorithms for solving any string related question we need to analyze the time and space complexity of a particular operation performed on a string.
Operations performed in a string | Worst Case Complexity | Average Case Complexity | Best Case Complexity |
Access operation in string data structure | O ( 1 ) | O ( 1 ) | O ( 1 ) |
Delete operation in string data structure | O ( n ) | O ( n ) | O ( 1 ) |
Insert operation in string data structure | O ( n ) | O ( n ) | O ( 1 ) |
Searching operation in string | O ( n * m ) | O ( n ) | O ( 1 ) |
Concatenation | O ( n + m ) | O ( n + m ) | O ( n ) |
Application of String in Data Structure
Checking valid database information
String can be used to check whether the given information of a user is valid or not in a database.
Used in string searching and pattern matching algorithm
Substring searching algorithms like Knuth-Morris-Pratt (KMP) , Robin- Karp and Boyer-Moore use string for text processing or efficient searching.
Strings are also used in pattern matching or validating strings and if we want to extract any phone number also.
Strings in Natural Language Processing
Tokenization and sentiment analysis heavily rely on manipulation that can only be done through the use of string data structure.
Advantages of String Data Structure
Easy to use
Strings are easy to implement and we can easily reverse , concatenate and insert strings anywhere. Syntax or implementation of strings are easy in comparison to other data structures that easily goes with the skill set of programmers.
Representation of data types and formats
We can easily represent other data types with the help of strings like date and time format HH : MM, we can also cover the format of date and year DD : MM : YYYY in the same format.
Including time with hours also HH : MM : SS
Disadvantages of String
More memory consumption
String is more memory consuming, like if we are using long string or text then it is more memory consumed and takes large spaces when we use it with mobile phones or tablets etc.
Security Exposure
Strings are more security vulnerable because if we are using long strings and there is a loophole remaining then the attackers easily change the text of the long string or may add some malicious code to it.
Conclusion
In this blog we have covered almost everything that includes the operations performed on the strings, how strings are actually represented in data structures when it comes to managing and storing the data efficiently. Covering practical examples while doing operations on it. String is a versatile concept that covers a lot of real time applications.